What Intcount Value Causes the Loop in Review Question 7 to End?
4.1. While Loops¶
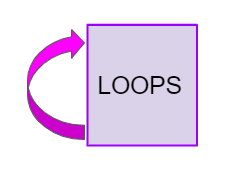
When you play a song, you can set it to loop, which ways that when information technology reaches the terminate it starts over at the starting time. A loop in programming, besides called iteration or repetition, is a way to repeat i or more statements. If you didn't have loops to allow you to repeat code, your programs would become very long very quickly! Using a sequence of code, selection (ifs), and repetition (loops), the control structures in programming, you can construct an algorithm to solve almost any programming problem!
A while
loop executes the body of the loop as long as (or while) a Boolean condition is true. When the status is false, we get out the loop and continue with the statements that are after the body of the while
loop. If the condition is false the first time yous check it, the body of the loop will not execute.
Notice the while
statement looks a lot like an if
statement, simply it runs more than than once. The curly brackets { } are optional when there is just 1 argument post-obit the condition, just required if there are more than than 1 statement in the loop. In the AP examination, they will always use curly brackets, which is a expert practice to follow.
// if statements just run once if the condition is true if ( condition ) { statements ; } // while statements are repeated while the condition is truthful while ( condition ) { statements ; }
If you took AP CSP with a block programming language like App Inventor, you lot may have used a loop block similar below that looks very similar to Java while loops (or you may have used a for loop which volition be covered in the next lesson). Almost every programming language has a while loop.

Figure ane: Comparing App Inventor and Coffee for while loops¶
If y'all're used to a language like Snap! or Scratch, you may be familiar with the Repeat Until loop. Nonetheless, you have to exist very conscientious comparing echo until to while loops. The while examination is the contrary of the repeat until test. For example, if you are repeatedly moving until reaching x position 100, y'all must create a Java while loop that repeatedly moves while information technology has not even so reached x position 100 or is less than 100 as below.
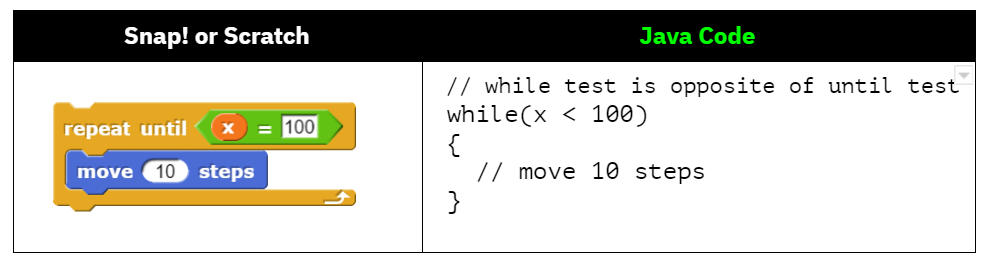
Figure 2: Comparing Snap! or Scratch Repeat Until Loop to Java while loop¶
The following video introduces while loops.
Here'due south what the flow of command looks like in a Java while loop. Notice that while the condition is true, the loop body is repeated.
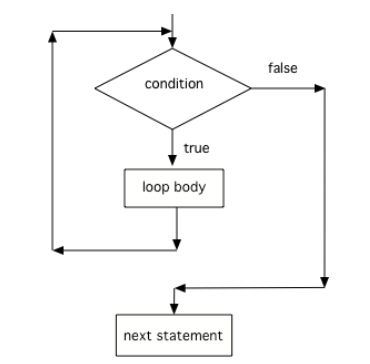
Effigy three: Control Flow in a while Loop¶
iv.1.1. Three Steps to Writing a Loop¶
The simplest loops are counter-controlled loops like beneath, where the loop command variable is a counter that controls how many times to repeat the loop. There are 3 steps to writing a loop using this loop control variable as seen below in a loop that counts from i to ten.
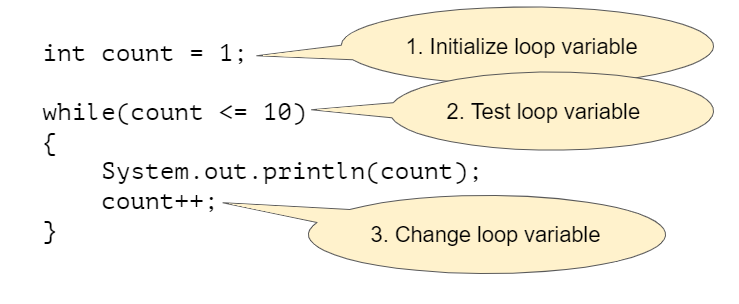
Effigy 4: Three Steps of Writing a Loop¶
Note
Remember these iii steps to writing a loop:
-
Initialize the loop variable (before the while loop)
-
Examination the loop variable (in the loop header)
-
Change the loop variable (in the while loop body at the cease)
Coding Exercise
Hither is a while loop that counts from 1 to v that demonstrates the 3 steps of writing a loop. Can you modify information technology to count from two to 10?
Java doesn't require your code to be correctly indented (code moved to the correct a few spaces) to get in clear what statements are role of the torso of the loop, simply it is standard practice to exercise so.
Note
On the free response role of the test, the reader will use the indention when determining the meaning of your code, fifty-fifty if yous forget the open or shut curly brace.
Bank check your agreement
- while (count == ten)
- This would not print out anything because count = 0 at the commencement of the loop, so information technology never equals 10.
- while (count < ten)
- This would print out 0 2 four vi eight. Try it in the Active Lawmaking window above.
- while (count <= 10)
- Yes, effort information technology in the Active Code window in a higher place.
- while (count > x)
- This would not impress out anything because count = 0 at the start of the loop, so it is not greater than 10.
four-1-iii: Consider the post-obit code segment. Which of the following tin be used as a replacement for the missing loop header so that the loop prints out "0 two 4 6 8 10"?
int count = 0 ; /* missing loop header */ { Organisation . out . print ( count + " " ); count += 2 ; }
4.one.ii. Tracing Loops¶
A really important skill to develop is the power to trace the values of variables and how they alter during each iteration of a loop.
Y'all tin can create a tracing table that keeps track of the variable values each fourth dimension through the loop as shown beneath. This is very helpful on the test. Studies accept shown that students who create tables similar this do much better on lawmaking tracing issues on multiple choice exams.
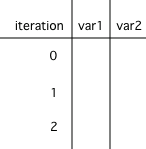
Figure five: A trace table showing the values of all of the variables each time through the loop. Iteration 0 means earlier the loop.¶
Scout the post-obit video for a tracing demo. When you lot are tracing through lawmaking, pretend to be the estimator running the code line by line, repeating the code in the loop, and keeping rails of the variable values and output.
Check your agreement
- 0
- Count is changed inside the loop and after the loop.
- 1
- Count is changed inside the loop and after the loop.
- 16
- Don't forget to decrease 10 from count subsequently the loop.
- vi
- Yes, the loop volition keep multiplying count by 2 to get ii, 4, 8, 16 and so it subtracts 10 from sixteen afterwards the loop.
4-1-5: Consider the following code segment. What is count'south value after running this lawmaking segment? (To trace through the lawmaking, keep track of the variable count and its value through each iteration of the loop.)
int count = one ; while ( count <= x ) { count *= 2 ; } count = count - 10 ;
Stride through the code higher up with the visualizer.
- 5 iv 3 2 one
- ten is initialized (fix) to -five to beginning.
- -5 -4 -3 -2 -1
- ten is incremented (x++) earlier the print statement executes.
- -4 -3 -two -ane 0
- 10 is set to -5 to start but then incremented by 1 so it beginning prints -iv.
4-1-half dozen: What does the following code print? (To trace through the lawmaking, continue rails of the variable ten and its value, the iteration of the loop, and the output every time through the loop.)
int x = - 5 ; while ( x < 0 ) { 10 ++ ; System . out . print ( x + " " ); }
4.1.3. Common Errors with Loops¶
One common error with loops is infinite loops. An infinite loop is i that never stops (the condition is always true).
// an infinite loop while ( true ) { System . out . println ( "This is a loop that never ends" ); }
The infinite loop in a higher place is pretty obvious. But, most infinite loops are adventitious. They unremarkably occur because you lot forget to modify the loop variable in the loop (step 3 of a loop).
Another common error with loops is an off-by-i error where the loop runs one too many or one besides few times. This is usually a trouble with pace two the exam condition and using the incorrect relational operator < or <=.
Coding Exercise
The while loop should print out the numbers ane to viii, merely it has 2 errors that crusade an space loop and an off-past-one error. Can you lot gear up the errors? If you run an space loop, you lot may demand to refresh the folio to cease information technology (and then make sure all active code windows on the page have been saved and click on Load History afterwards refreshing).
4.1.iv. Input-Controlled Loops¶
Yous can use a while
loop to repeat the body of the loop a certain number of times every bit shown to a higher place. However, a while
loop is typically used when you don't know how many times the loop will execute. It is oft used for a input-controlled loop where the user'due south input indicates when to terminate. For example, in the Magpie chatbot lab on repl.it below, the while loop stops when you type in "Bye". The stopping value is oft called the sentinel value for the loop. Find that if you type in "Good day" right away, the loop will never run. If the loop condition evaluates to false initially, the loop body is not executed at all. Another manner to stop the loop prematurely is to put in a return statement that makes it immediately render from the method.
Coding Exercise
Here's another example with numbers on repl.it. This lawmaking calculates the average of positive numbers, but information technology is missing the condition for the loop on line fourteen. Permit'southward utilise -1 as the sentinel value. Add the status to the while loop to run while the user does not input -1. What would happen if you forgot step iii (change the loop variable - go a new input)? Try commenting out line 19 with // to see what happens (note at that place is a stop button at the top!).
There are standard algorithms that use loops to compute the sum or boilerplate similar above, or decide the minimum or maximum value entered, or the frequency of a sure condition. You can also utilise loops to place if some integers are evenly divisible past other integers or identify the individual digits in an integer. We will see a lot more of these algorithms in Unit 6 with loops and arrays.
four.1.5.
Programming Challenge : Guessing Game¶
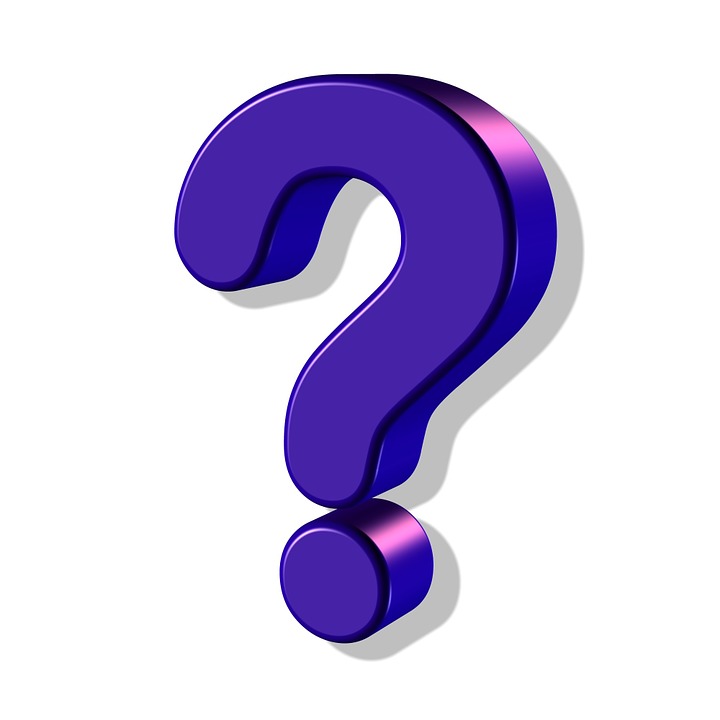
Nosotros encourage you to work in pairs on this guessing game. In the guessing game, the reckoner picks a random number from 0-100 and you have to guess it. After each estimate, the computer will requite you clues like "Too loftier" or "Too low". Here's the pseudocode for the guessing game. Pseudocode is an English description or plan of what your code will practise footstep by step. What's the loop variable for this program? Tin can you identify the three steps of writing this loop with respect to the loop variable?
-
Choose a random number from 0-100
-
Get the start gauge
-
Loop while the judge does not equal the random number,
-
If the gauge is less than the random number, print out "Too low!"
-
If the guess is greater than the random number, print out "Also high!"
-
Get a new guess (save it into the same variable)
-
-
Print out something like "You lot got it!"
Every bit an extension to this project, you lot tin can add together a counter variable to count how many guesses the user took and print it out when they guess correctly.
When yous stop and run your programme, what is a good guessing strategy for guessing a number between 0 and 100? What was your commencement guess? One great strategy is to always divide the guessing space into two and eliminating half, so guessing 50 for the outset gauge. This is called a separate and conquer or binary search algorithm. If your guess is between 0-100, you should exist able to judge the number within seven guesses. Another extension to this challenge is to examination whether the user got it in 7 guesses or less and provide feedback on how well they did.
For this project, you will need to use the Scanner class for input and repl.it or another IDE of your choice.
Copy and paste all of your code from your repl.information technology and run to come across if it passes the autograder tests. Include the link to your repl.it lawmaking in comments. Note that this lawmaking will only run with the autograder'due south input and volition not ask the user for input.
4.1.6. Summary¶
-
Iteration statements (loops) change the flow of control by repeating a ready of statements zero or more times until a condition is met.
-
Loops often take a loop control variable that is used in the boolean status of the loop. Call back the three steps of writing a loop:
-
Initialize the loop variable
-
Test the loop variable
-
Change the loop variable
-
-
In loops, the Boolean expression is evaluated before each iteration of the loop body, including the outset. When the expression evaluates to true, the loop trunk is executed. This continues until the expression evaluates to simulated which signals to exit the loop. If the Boolean expression evaluates to faux initially, the loop torso is not executed at all.
-
A loop is an infinite loop when the Boolean expression e'er evaluates to true so that the loop never ends.
-
Off by ane errors occur when the iteration argument loops one time too many or one fourth dimension likewise few.
-
If the Boolean expression evaluates to faux initially, the loop body is non executed at all.
-
Input-controlled loops oftentimes utilise a sentinel value that is input past the user like "bye" or -1 as the status for the loop to end. Input-controlled loops are not on the AP CS A exam, only are very useful to take data from the user.
-
At that place are standard algorithms to compute a sum or boilerplate.
You take attempted of activities on this page
shafferdentrejecome.blogspot.com
Source: https://runestone.academy/ns/books/published/csawesome/Unit4-Iteration/topic-4-1-while-loops.html
0 Response to "What Intcount Value Causes the Loop in Review Question 7 to End?"
Postar um comentário